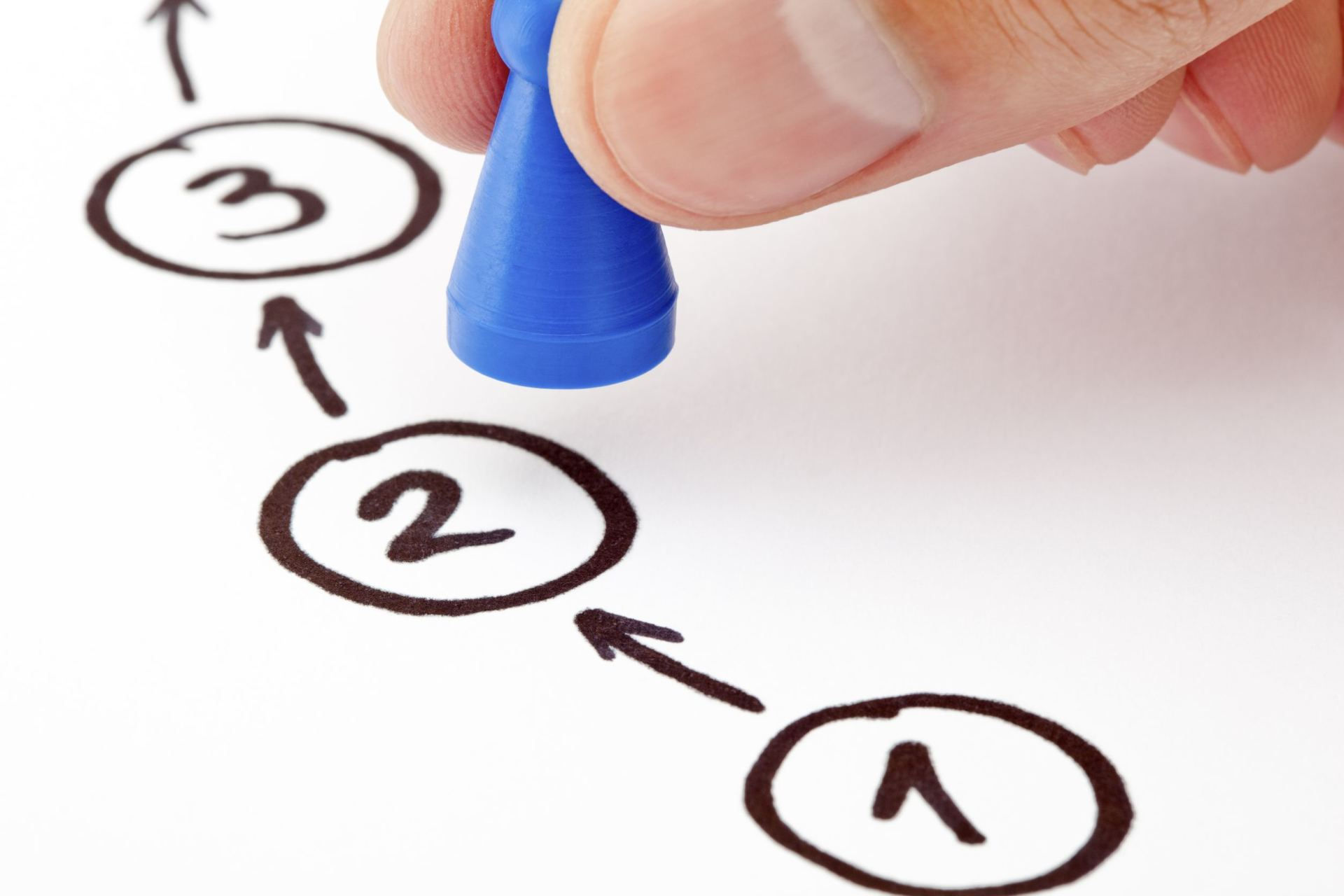
CLASE 11:
ORDENAMIENTO DE NUMEROS CON VECTORES Y FUNCIONES SIN PARAMETROS
1) CODIGO DE PROGRAMACION
#include <iostream>
#include <math.h>
using namespace std;
//ZONA PUBLICA DE DECLARACIONES
int BURBUJA();
int SELECCION_SORT();
int INSERCION();
int SHELL();
int QUICK_SORT();
//DECLARACIONES
int Aux,i,p,q,r,N,j,A[6],B[6],C[6],D[6],E[6],May[6],Men[6],opcion, a,b,c,m,n,izq,der;
int Min,pos;
bool band;
int main()
{
cout<<" MENU "<<endl;
cout<<"***********************************"<<endl;
cout<<"1) BURBUJA"<<endl;
cout<<"2) SELECCION"<<endl;
cout<<"3) INSERCION"<<endl;
cout<<"4) SHELL"<<endl;
cout<<"5) QUICK SORT"<<endl;
cout<<"SELECCIONE UNA OPCION: "<<endl;
cin>>opcion;
cout<<endl;
switch(opcion)
{
case 1:
{
//EJERCICIO 1
cout<<"USTED ESCOGIO:"<<endl;
cout<<"*****METODO DE BURBUJAS*****"<<endl;
BURBUJA();
};break;
case 2:
{
//EJERCICIO 2
cout<<"USTED ESCOGIO:"<<endl;
cout<<"*****METODO POR SELECCION******"<<endl;
SELECCION_SORT();
};break;
case 3:
{
//EJERCICIO 3
cout<<"USTED ESCOGIO:"<<endl;
cout<<"*****METODO POR INSERCION*******"<<endl;
INSERCION();
};break;
case 4:
{
//EJERCICIO 4
cout<<"USTED ESCOGIO:"<<endl;
cout<<"******METODO SHELL*******"<<endl;
SHELL();
};break;
case 5:
{
//EJERCICIO 5
cout<<"USTED ESCOGIO:"<<endl;
cout<<"*******METODO QUICK SORT*******"<<endl;
QUICK_SORT();
};break;
}
}
//FIN DEL PROGRAMA
// ZONA DE FUNCIONES
int BURBUJA()
{
cout<<"******ESCRIBIENDO LOS ELEMENTOS DEL VECTOR******"<<endl;
cout<<"Ingrese el Numero de Elementos:"; cin>>N;
//ESCRIBIR LOS NUMEROS EN EL VECTOR
for (p=1;p<=N;p++)
{
cout<<"Ingrese el Vector A["<<p<<"]=";cin>>A[p];
}
cout<<endl;
//MIRAR VECTOR
cout<<"******MIRANDO LOS ELEMENTOS DEL VECTOR******"<<endl;
for(q=1;q<=N;q++)
{
cout<<"El Elemento del Vector A["<<q<<"]=";cout<<A[q]<<endl;
}
cout<<endl;
//ORDENAMIENTO DEL VECTOR POR BURBUJA
//Error el Mayor Numero es reemplazado por 0
for (i>0;i<=N-1;i++)//NUMERO DE REORDENAMIENTO
{
for(j=1;j<=N;j++)
{
pos=j;
Aux=A[j];
if(Aux>A[pos+1])
{
Aux=A[pos];
A[pos]=A[pos+1];
A[pos+1]=Aux;
}
else
{
A[pos] = Aux;
}
}
}
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........ORDENANDO NUMEROS........"<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPLETADO..........."<<endl;
cout<<endl;
cout<<"******MIRANDO EL NUEVO ORDEN DE LOS ELEMENTOS DEL VECTOR******"<<endl;
//MIRAR ORDENAMIENTO DEL VECTOR
for(r=1;r<=N;r=r+1)
{
cout<<"EL Nuevo Orden del Vector es:"<<A[r]<<endl;
}
}
int SELECCION_SORT()
{
cout<<"******ESCRIBIENDO LOS ELEMENTOS DEL VECTOR******"<<endl;
cout<<"Ingrese el Numero de Elementos:"; cin>>N;
//ESCRIBIR LOS NUMEROS EN EL VECTOR
for (p=1;p<=N;p=p+1)
{
cout<<"Ingrese el Vector B["<<p<<"]=";cin>>B[p];
}
cout<<endl;
//MIRAR VECTOR
cout<<"******MIRANDO LOS ELEMENTOS DEL VECTOR******"<<endl;
for(q=1;q<=N;q=q+1)
{
cout<<"El Elemen o del Vector B["<<q<<"]=";cout<<B[q]<<endl;
}
cout<<endl;
//ORDENAMIENTO POR SELECCION DEL VECTOR
//Buscando el Minimo Elemento del Vector
for(i=0;i<=N;i++)
{
Min=i;
for(j=i+1;j<=N;j++)
{
if(B[j] < B[Min])
{
Min = j;
}
}
Aux = B[i];
B[i] = B[Min];
B[Min] = Aux;
}
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........BUSCANDO MINIMOS........"<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........ORDENANDO NUMEROS........"<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPLETADO..........."<<endl;
cout<<endl;
//MIRAR ORDENAMIENTO DEL VECTOR
for(r=1;r<=N;r=r+1)
{
cout<<"EL Nuevo Orden del Vector es:"<<B[r]<<endl;
}
}
int INSERCION()
{
cout<<"******ESCRIBIENDO LOS ELEMENTOS DEL VECTOR******"<<endl;
cout<<"Ingrese el Numero de Elementos:"; cin>>N;
//ESCRIBIR LOS NUMEROS EN EL VECTOR
for (p=1;p<=N;p++)
{
cout<<"Ingrese el Vector C["<<p<<"]=";cin>>C[p];
}
cout<<endl;
//MIRAR VECTOR
cout<<"******MIRANDO LOS ELEMENTOS DEL VECTOR******"<<endl;
for(q=1;q<=N;q++)
{
cout<<"El Elemento del Vector C["<<q<<"]=";cout<<C[q]<<endl;
}
cout<<endl;
//ORDENAMIENTO POR INSERCION DEL VECTOR
for(i=0;i<=N;i++)
{
pos = i; //posicion de Arreglo guardado en pos
Aux = C[i];//Numero de La Posicion del Arreglo Guardado en Auxiliar
while((pos>0) && (C[pos-1] > Aux))//Posicion mayora 0 y Numero de Posicion anterior mayor a Auxiliar
{
C[pos] = C[pos-1];//Numero de Posicion anterior Reemplaza Numero de Posicion Actual
pos--;//Posicion anterior igual a Posicion Actual
}
C[pos] = Aux;//Numero Auxiliar conserva el numero de Posicion Actual
}
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPARANDO NUMEROS........."<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........ORDENANDO NUMEROS........"<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPLETADO..........."<<endl;
cout<<endl;
//MIRAR ORDENAMIENTO DEL VECTOR
for(r=1;r<=N;r++)
{
cout<<"EL Nuevo Orden del Vector es:"<<C[r]<<endl;
}
}
int SHELL()
{
cout<<"******ESCRIBIENDO LOS ELEMENTOS DEL VECTOR******"<<endl;
cout<<"Ingrese el Numero de Elementos:"; cin>>N;
//ESCRIBIR LOS NUMEROS EN EL VECTOR
for (p=1;p<=N;p=p+1)
{
cout<<"Ingrese el Vector D["<<p<<"]=";cin>>D[p];
}
cout<<endl;
//MIRAR VECTOR
cout<<"******MIRANDO LOS ELEMENTOS DEL VECTOR******"<<endl;
for(q=1;q<=N;q=q+1)
{
cout<<"El Elemento del Vector D["<<q<<"]=";cout<<D[q]<<endl;
}
cout<<endl;
//ORDENAMIENTO POR SHELL DEL VECTOR
//Error El Numero menor es reemplazado por un 0
for(i=0;i<=N;i++)
{
pos=i;
while (pos>1)
{
pos=(pos/2);
band=true;
while (band==true)
{
band=false;
j=0;
while ((j+pos)<=i)
{
if(D[j]>D[j+pos])
{
Aux=D[j];
D[j]=D[j+pos];
D[j+pos]=Aux;
band=true;
}
j++;
}
}
}
}
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPARANDO NUMEROS........."<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........ORDENANDO NUMEROS........"<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPLETADO..........."<<endl;
cout<<endl;
//MIRAR ORDENAMIENTO DEL VECTOR
for(r=1;r<=N;r=r+1)
{
cout<<"EL Nuevo Orden del Vector es:"<<D[r]<<endl;
}
}
int QUICK_SORT()
{
cout<<"******ESCRIBIENDO LOS ELEMENTOS DEL VECTOR******"<<endl;
cout<<"Ingrese el Numero de Elementos:"; cin>>N;
//ESCRIBIR LOS NUMEROS EN EL VECTOR
for (p=1;p<=N;p=p+1)
{
cout<<"Ingrese el Vector E["<<p<<"]=";cin>>E[p];
}
cout<<endl;
//MIRAR VECTOR
cout<<"******MIRANDO LOS ELEMENTOS DEL VECTOR******"<<endl;
for(q=1;q<=N;q=q+1)
{
cout<<"El Elemento del Vector E["<<q<<"]=";cout<<E[q]<<endl;
}
cout<<endl;
//ORDENAMIENTO POR QUICK SORT DEL VECTOR
for(i=0;i<=N;i++)
{
a=1;
Men[a]=1;
May[a]=N;
while (a>0)
{
m=Men[a];
n= May[a];
a--;
//Inicio Reduce Iterativo
izq=m;
der=n;
pos=m;
band=true;
while(band==true)
{
while((E[pos]<=E[der]) && (pos!=der))
{
der--;
}
if(pos==der)
{
band=false;
}
else
{
Aux=E[pos];
E[pos]=E[der];
E[der]=Aux;
pos=der;
}
while((E[pos]>=E[izq]) &&(pos!=izq))
{
izq++;
}
if(pos==izq)
{
band=false;
}
else
{
Aux=E[pos];
E[pos]=E[izq];
E[izq]=Aux;
pos=izq;
}
}
//Fin de Reduce Iterativo
if (m<(pos-1))
{
a++;
Men[a]=m;
May[a]=pos-1;
}
if (n>(pos+1))
{
a++;
Men[a]=pos+1;
May[a]=n;
}
}
}
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPARANDO NUMEROS........."<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........ORDENANDO NUMEROS........"<<endl;
cout<<endl;
cout<<endl;
cout<<endl;
cout<<"...........COMPLETADO..........."<<endl;
cout<<endl;
//MIRAR ORDENAMIENTO DEL VECTOR
for(r=1;r<=N;r=r+1)
{
cout<<"EL Nuevo Orden del Vector es:"<<E[r]<<endl;
}
}
2) PRUEBA DE CODIGO
* MENU:
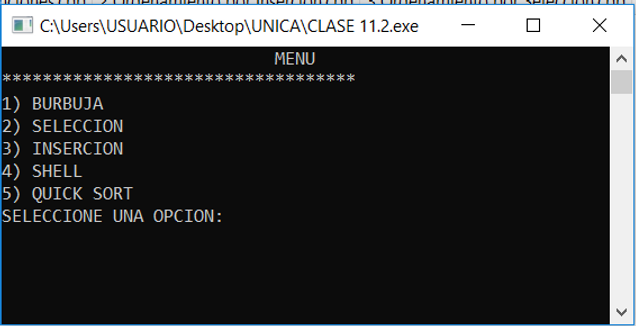
OPERACION 1: METODO DE ORDENAMIENTO "BURBUJA"

OPERACION 2: METODO DE ORDENAMIENTO "POR SELECCION"
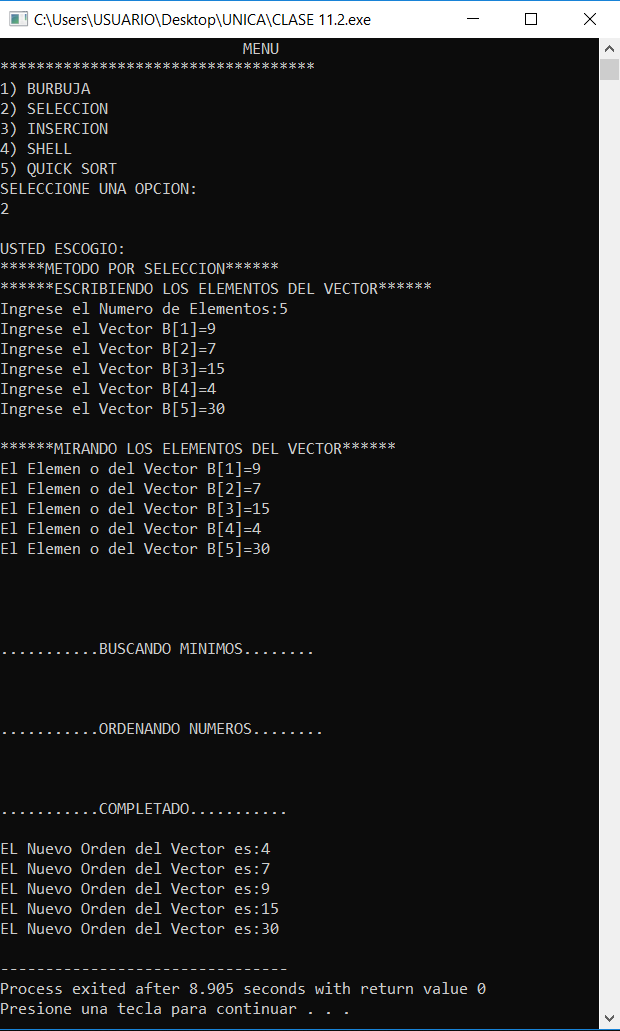
OPERACION 3: METODO DE ORDENAMIENTO "POR INSERCION"
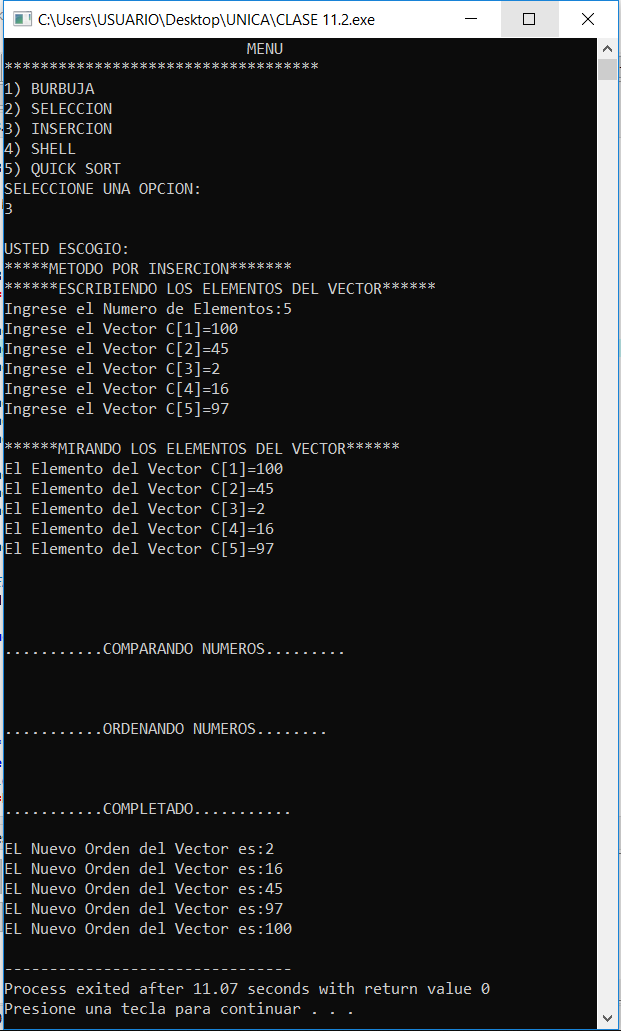
OPERACION 4: METODO DE ORDENAMIENTO "SHELL"
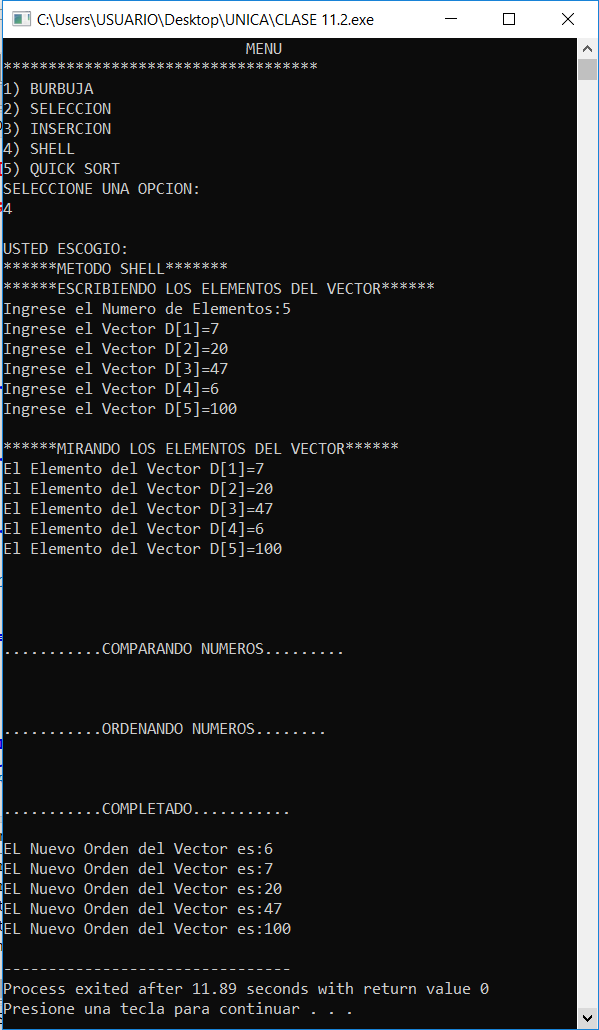
OPERACION 5: METODO DE ORDENAMIENTO "QUICK SORT"
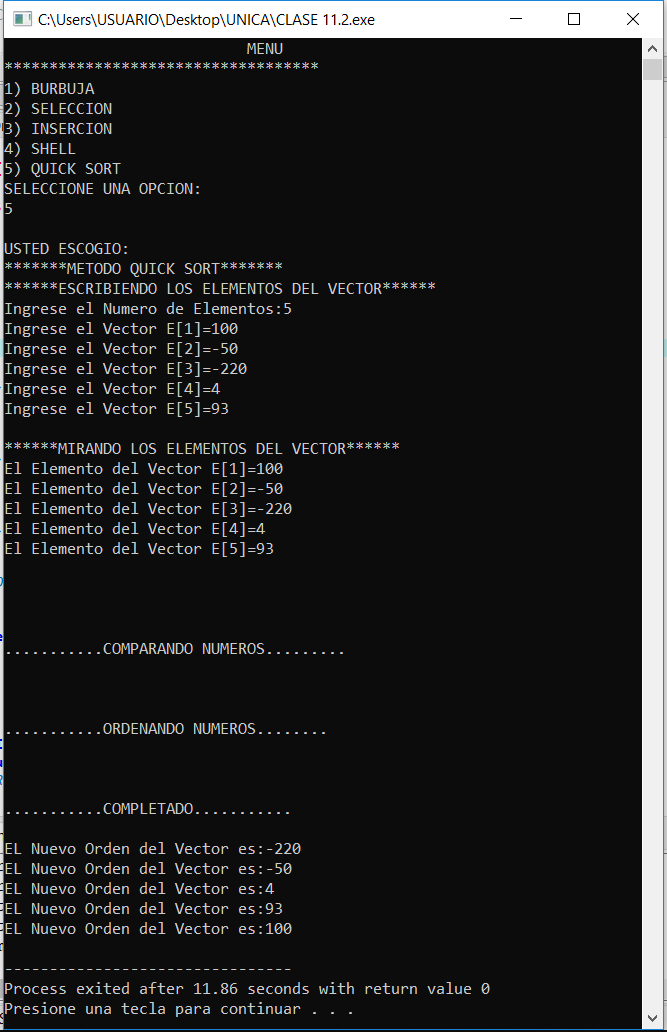